Verilog 学习笔记
Syntax
By default, Verilog simulators treat numbers as decimals.
4'hA = 4'd10 = 4'b1010 = 4'o12 // Decimal 10 can be represented in any of the four formats |
Numbers without a base_format specification are decimal numbers by default. Numbers without a size specification have a default number of bits depending on the type of simulator and machine.
integer a = 5423; // base format is not specified, a gets a decimal value of 5423 |
A sequence of characters enclosed in a double quote " "
is called a string. It cannot be split into multiple lines and every character in the string take 1-byte to be stored.
"Hello World!" // String with 12 characters -> require 12 bytes |
Data Types
There are different types of nets each with different characteristics, but the most popular and widely used net in digital designs is of type wire
.
It is illegal to redeclare a name already declared by a net, parameter or variable as shown in the code below.
module design; |
An integer
is a general purpose variable of 32-bits
integer count; // Count is an integer value > 0 |
Verilog data-type reg
can be used to model hardware registers since it can hold values between assignments. Note that a reg
need not always represent a flip-flop because it can also be used to represent combinational logic.
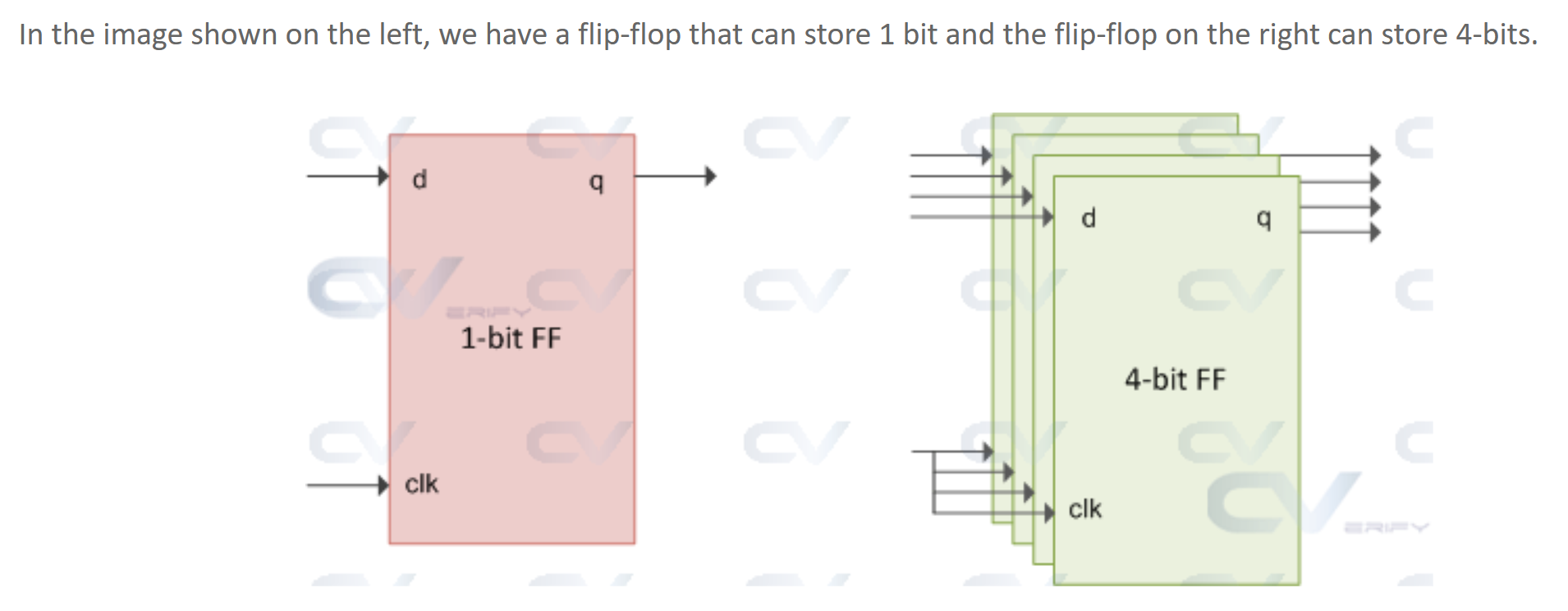
A time
variable is unsigned, 64-bits wide and can be used to store simulation time quantities for debugging purposes. A realtime
variable simply stores time as a floating point quantity.
time end_time; // end_time can be stored a time value like 50ns |
Scalar and Vector
A net or reg
declaration without a range specification is considered 1-bit wide and is a scalar. If a range is specified, then the net or reg
becomes a multibit entity known as a vector.
wire o_nor; // single bit scalar net |